from StackOverflow: update some specific field of an entity
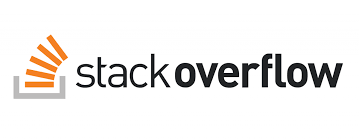
I take an old answer about the Room usage from stack overflow. The original post is:
I am using android room persistence library for my new project. I want to update some field of the table. I have tried like in my Dao
–
// Method 1:
@Dao
public interface TourDao {
@Update
int updateTour(Tour tour);
}
But when I try to update using this method then it updates every field of the entity where it matches the primary key value of tour object. I have used @Query
// Method 2:
@Query("UPDATE Tour SET endAddress = :end_address WHERE id = :tid")
int updateTour(long tid, String end_address);
It is working but there will be many queries in my case because I have many fields in my entity. I want to know how can I update some field (not all) like Method 1
where id = 1; (id is the auto generate primary key).
// Entity:
@Entity
public class Tour {
@PrimaryKey(autoGenerate = true)
public long id;
private String startAddress;
private String endAddress;
//constructor, getter and setter
}
On stackoverflow, you will find find the answer using Room library. Here we solve the same problem using Kripton Persistence Library. To do this, you need just to define the DAO interface, the entity and the datasource:
@BindDao(Tour.class)
public interface TourDao {
@BindSqlUpdate(where = "id=:{id}")
int updateTour(String startAddress, long id);
@BindSqlUpdate(where = "id=:{id}")
int updateTour2(String endAddress, long id);
@BindSqlSelect(jql = "select * from tour")
List<Tour> getList();
}
@BindType
public class Tour {
public long id;
public String startAddress;
public String endAddress;
}
@BindDataSource(daoSet = { TourDao.class }, fileName = "tour.db")
public interface TourDataSource {
}
As you can see from DAO definition: to define an update SQL that updates only one field using a where condition, you simply need to define the SQL where condition. Kripton will detect which method’s parameter is used to update the table and which is used to define the where conditions (because it is used the annotation’s attribute where
)
For more information about Kripton visit the github repository.