Kripton ORM: how to store a HashSet in a column
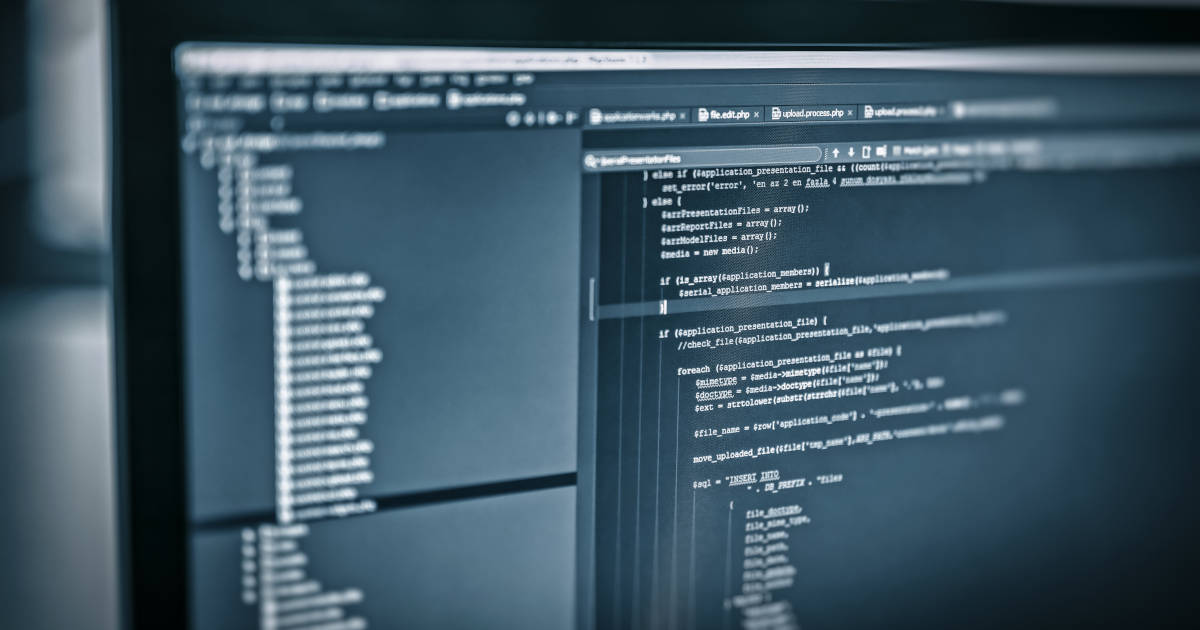
Kripton ORM is the Kripton’s module to manage SQLite database. Store a HashSet in a column is quite easy. Let’s suppose we have a Java model mapped to the SQLite database in the following way:
@BindTable(name = "SchoolLunches")
public class SchoolLunch {
@BindColumn(columnType=ColumnType.PRIMARY_KEY)
private long lunchId;
public long getLunchId() {
return lunchId;
}
public void setLunchId(long lunchId) {
this.lunchId = lunchId;
}
private boolean fresh;
public boolean isFresh() {
return fresh;
}
public void setFresh(boolean fresh) {
this.fresh = fresh;
}
private boolean containsMeat;
private HashSet fruits;
public boolean isContainsMeat() {
return containsMeat;
}
public void setContainsMeat(boolean containsMeat) {
this.containsMeat = containsMeat;
}
public HashSet getFruits() {
return fruits;
}
public void setFruits(HashSet fruits) {
this.fruits = fruits;
}
}
The associated DAO interface:
@BindDao(value=SchoolLunch.class)
public interface SchoolLunchDAO {
@BindSqlSelect
List getAll();
@BindSqlInsert
void insertAll(SchoolLunch schoolLunches);
@BindSqlDelete
void deleteAll();
}
And the database definition:
@BindDataSource(daoSet={SchoolLunchDAO.class}, fileName = "school.db")
public interface SchoolLunchDataSource {
}
Store a HashSet is simple as define it in the SchoolLunch
. Kripton will generate from DAO interface the implementation and the associated table class.
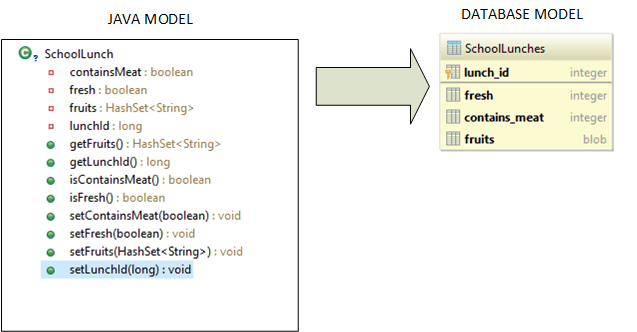
Kripton allows to persist every collection of String, Long, Short and every Kripton supported kind. Collections are stored in JSON format.
This is a JUnit test to see how to integrate all we see in this article:
@Test
public void test() {
HashSet fruitSet = new HashSet<>();
fruitSet.add("Apple");
fruitSet.add("Orange");
SchoolLunch schoolLunch = new SchoolLunch();
schoolLunch.setContainsMeat(false);
schoolLunch.setFresh(true);
schoolLunch.setFruits(fruitSet);
BindSchoolLunchDataSource dataSource=BindSchoolLunchDataSource.instance();
dataSource.openWritableDatabase();
SchoolLunchDAOImpl schoolLunchDAO = dataSource.getSchoolLunchDAO();
schoolLunchDAO.insertAll(schoolLunch);
List schoolLunches = schoolLunchDAO.getAll();
assertEquals(schoolLunches.size(), 1);
SchoolLunch extractedSchoolLunch = schoolLunches.get(0);
assertEquals(false, extractedSchoolLunch.isContainsMeat());
assertEquals(true, extractedSchoolLunch.isFresh());
assertEquals(2, extractedSchoolLunch.getFruits().size());
dataSource.close();
}
The generated log is:
I/AbstractDataSource$2, onCreate (line 223): Create database 'school.db' version 1
I/AbstractDataSource$2, onCreate (line 223): DDL: CREATE TABLE SchoolLunches (lunch_id INTEGER PRIMARY KEY AUTOINCREMENT, fresh INTEGER, contains_meat INTEGER, fruits BLOB);
I/TestStack44633883Runtime, test (line 46): database OPEN READ_AND_WRITE_OPENED (connections: 1)
I/TestStack44633883Runtime, test (line 50): INSERT INTO SchoolLunches (contains_meat, fresh, fruits) VALUES (:contains_meat, :fresh, :fruits)
I/TestStack44633883Runtime, test (line 50): ==> :contains_meat = 'false' (java.lang.Boolean)
I/TestStack44633883Runtime, test (line 50): ==> :fresh = 'true' (java.lang.Boolean)
I/TestStack44633883Runtime, test (line 50): ==> :fruits = '{"element":["Apple","Orange"]}' (byte[])
I/TestStack44633883Runtime, test (line 52): SELECT lunch_id, fresh, contains_meat, fruits FROM SchoolLunches
I/CursorWindowStats: Created a new Cursor. # Open Cursors=1 (# cursors opened by this proc=1)
D/SQLiteCursor: received count(*) from native_fill_window: 1
I/TestStack44633883Runtime, test (line 52): Rows found: 1
I/TestStack44633883Runtime, test (line 60): database CLOSED (READ_AND_WRITE_OPENED) (connections: 0)
This post was inspired by the following post on StackOverflow.
If you like Kripton does not hesitate to give it a star on GitHub.
xcesco